Sliders with feedback and without anchoring
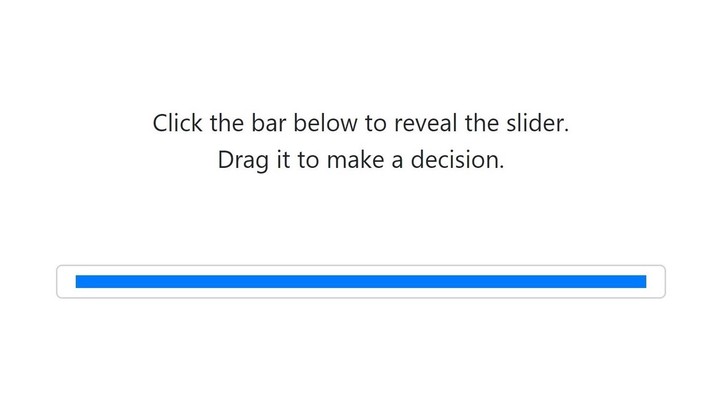
Many experiments, laboratory studies, and surveys use sliders rather than radiobuttons or dropdown menus to elicit information from participants. One of the advantages of sliders is that enable us to give participants real-time feedback on their input while preventing them from anchoring too strongly on the possible input range and starting location of the slider. In this post, I share a few lines of codes that help you program such sliders.
EDIT: Max R. P. Grossmann added an updated version of the code below that works well with newer versions of oTree, different browsers, and mobile devices. Check out his code here.
Basic Code for a Slider
The HTML code for a slider uses <input>
with type="range"
. I share the code for a slider that ranges from 0 through 10 with steps of 1 below.
<input type="range" name="slider_one" value="None" step="1" style="width:500px" min="0" max="10" id="id_slider_one" class="form-control">
Adding Real-time Feedback
Since sliders give participants a natural feeling of control over the input, we can combine it with feedback that updates according to the input they have selected with the slider. To add real-time feedback, we add two lines of HTML code. First, we add the line of HTML code below and position it just above the HTML code for the slider we just made.
<p id="feedback_one"><br></p>
This HTML code will contain the feedback. However, we also need to make sure that this HTML code updates depending on the input that the participant has selected on the slider. Thus, we also add the following Javascript code.
<script>
$(document).ready(function () {
$('input[name=slider_one]').on('input', function() {
document.getElementById("feedback_one").innerHTML = `You have selected `+$(this).val()+'!';
});
});
<\script>
The Javascript above will push the text “You have selected [value of slider_one]!” to the line of HTML code we previously added as soon as participants drag or change the slider.
Slider Input Check
I always include a check on the same page that checks whether the participant has actually touched the slider. In many cases, we want to make input mandatory. Thus, we need to find a way to prevent the participant from submiting the form until he or she has touched the slider. Such a check has three parts of code. First, you include another <input>
with type="hidden"
, which I locate above the HTML code for the slider and the HTML code for the feedback.
<input type="hidden" name="check_slider_one" value="" id="id_check_slider_one"/>
Note that this hidden input has no starting value. Next, you add the line $('#check_slider_one').val(1);
to the Javascript code we previously created:
<script>
$(document).ready(function () {
$('input[name=slider_one]').on('input', function() {
document.getElementById("feedback_one").innerHTML = `You have selected `+$(this).val()+'!';
$('#check_slider_one').val(1);
});
});
<\script>
This new line will change the hidden input from nothing to one as soon as the participant provides touches the slider. If we make this hidden input mandatory, then participants cannot continue unless they have touched the slider. If you are using oTree, you can include the following Python code in pages.py:
class slider_page(Page):
form_model = 'player'
form_fields = ['slider_one', 'check_slider_one']
def error_message(self, value):
if value["check_slider_one"] == None:
return 'Please use the slider to make a decision.'
Observe that I have also included the necessary information for the forms on the HTML page “slider_page.” The second part of the code will present participants with the message “Please use the slider to make a decision” if they have not touched the slider.
Preventing Anchoring
One of the disadvantages of sliders over other input forms is that the starting position of the slider on the bar may cause participants to anchor on that starting point. For instance, we can choose that the slider starts in the middle of the bar, which may cause participants to give answers around the middle of the slider range. We could also let the slider start at one of the endpoints, which may cause participants to skew their answers more strongly toward one of the endpoints. One way to prevent this issue is to hide the slider on the bar untill participants have clicked on the bar. This prevents participants from anchoring toward the starting point or endpoints of the slider. The first code we need to add is style code:
<style>
.myclass::-webkit-slider-thumb {
box-shadow: 1px 1px 1px #000000, 0px 0px 1px #007afe;
border: 1px solid #000000;
height: 21px !important;
width: 10px !important;
border-radius: 0px !important;
background: #ffffff !important;
cursor: pointer !important !important;
-webkit-appearance: none !important;
margin-top: -7px !important;
}
input[name=slider_one] {
-webkit-appearance: none;
margin: 18px 0;
width: 100%;
}
input[name=slider_one]:focus {
outline: none;
}
input[name=slider_one]::-webkit-slider-runnable-track {
width: 100%;
height: 8.4px;
cursor: pointer;
animate: 0.2s;
{#box-shadow: 1px 1px 1px #000000, 0px 0px 1px #0d0d0d;#}
background: #007afe;
border-radius: 0px;
border: 0.0px solid #ffffff;
}
input[name=slider_one]::-webkit-slider-thumb {
border: 0px;
height: 0px;
width: 0px;
border-radius: 0px;
-webkit-appearance: none;
}
</style>
This code redesigns the slider and its bar. It also helps us hide and show the slider. Take particular note of “slider_one” in this code because it refers to the name of your slider on the HTML page. Next, we extend the script we created earlier one more time to make sure the slider appears as soon as participants touch the bar.
<script>
$(document).ready(function () {
$('input[name=slider_one]').on('input change', function () {
$('input[name=slider_one]').addClass('myclass');
});
$('input[name=slider_one]').on('input', function() {
document.getElementById("feedback_one").innerHTML = `You have selected `+$(this).val()+'!';
$('#check_slider_one').val(1);
});
});
<\script>
The Final Result
If you combine all the code above, you get a magnificent slider with real-time feedback, without anchoring, and with a validation check.
Do you have tips or thoughts about improving the code? Or do you have other problems? Please comment below!
How to reference this online article?
Van Pelt, V. F. J. (2020, February 19). Sliders with feedback and without anchoring. Accounting Experiments, Available at: https://www.accountingexperiments.com/post/sliders/.